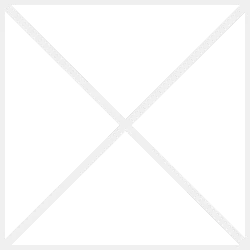
This ad doesn't have any photos.
|
Date | 2/25/2025 12:30:09 PM |
Want to learn how to count in C? Counting is an essential part of programming, whether it’s counting digits, iterations, or elements in an array. If you're new to C programming, understanding counting techniques can improve your logical thinking and coding efficiency.
One of the simplest counting programs in C involves determining the number of digits in a given number. Here's an example:
c Copy Edit #include
int main() { int num, count = 0; printf("Enter a number: "); scanf("%d", &num);
do { count++; num /= 10; } while (num != 0);
printf("Count of digits: %d\n", count); return 0; } In this program, a do-while loop ensures that at least one iteration occurs, making it efficient for handling the number 0.
Counting is also widely used in arrays. Here’s a basic example of counting even numbers in an array:
c Copy Edit #include
int main() { int arr[] = {2, 5, 7, 8, 10, 3}; int count = 0, size = sizeof(arr) / sizeof(arr[0]);
for (int i = 0; i < size; i++) { if (arr[i] % 2 == 0) count++; }
printf("Count of even numbers: %d\n", count); return 0; } This program iterates through an array and counts how many even numbers exist.
|