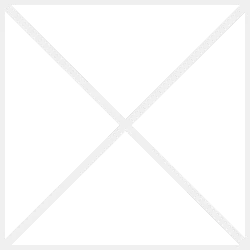
This ad doesn't have any photos.
|
Arrays are one of the most get value from array javascript, enabling developers to store and manipulate collections of data efficiently. Whether you're working with numbers, strings, or objects, retrieving values from an array is a crucial skill. This guide explores different techniques for accessing values from arrays in JavaScript, from basic index-based retrieval to advanced methods like map(), filter(), and find().
1. Accessing Array Values Using Indexing The simplest way to retrieve a value from an array is by using its index. JavaScript arrays are zero-based, meaning the first element is at index 0.
javascript Copy Edit let fruits = ["Apple", "Banana", "Cherry"]; console.log(fruits[0]); // Output: Apple console.log(fruits[2]); // Output: Cherry If you try to access an index that doesn't exist, JavaScript will return undefined.
2. Using at() Method Introduced in ES2022, the at() method provides a cleaner way to access elements, including support for negative indexing.
javascript Copy Edit console.log(fruits.at(-1)); // Output: Cherry This is useful for retrieving the last element without manually calculating the index.
3. Finding Values with find() If you're dealing with complex data structures, the find() method is handy. It returns the first element that meets a specified condition.
javascript Copy Edit let users = [ { id: 1, name: "Alice" }, { id: 2, name: "Bob" } ]; let user = users.find(user => user.id === 2); console.log(user.name); // Output: Bob 4. Retrieving Multiple Values with filter() The filter() method returns all elements that match a given condition.
javascript Copy Edit let numbers = [10, 25, 30, 45]; let largeNumbers = numbers.filter(num => num > 20); console.log(largeNumbers); // Output: [25, 30, 45] 5. Extracting Values Using map() The map() method is ideal for transforming arrays by extracting specific properties from objects.
javascript Copy Edit let names = users.map(user => user.name); console.log(names); // Output: ["Alice", "Bob"] 6. Getting the Last Element Dynamically To retrieve the last element dynamically, use:
javascript Copy Edit console.log(fruits[fruits.length - 1]); // Output: Cherry 7. Checking If a Value Exists with includes() The includes() method checks if an array contains a specific value.
javascript Copy Edit console.log(fruits.includes("Banana")); // Output: true Conclusion JavaScript provides multiple ways to retrieve values from an array, each suited for different use cases. Whether you use simple indexing, find(), filter(), or map(), understanding these techniques enhances your ability to manipulate and extract data effectively.
|