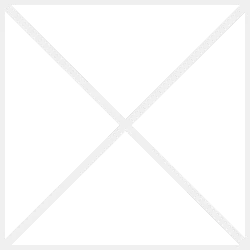
This ad doesn't have any photos.
|
JavaScript arrays are versatile and widely used for storing and manipulating collections of data. Extracting values from an array is a fundamental task, and get value from array javascript ways to achieve this. In this article, we will explore various techniques to get values from an array in JavaScript.
1. Accessing Values by Index
Each element in a JavaScript array has a zero-based index. You can retrieve values using bracket notation: let fruits = ["Apple", "Banana", "Cherry"]; console.log(fruits[0]); // Output: Apple console.log(fruits[1]); // Output: Banana console.log(fruits[2]); // Output: Cherry 2. Using the find() Method
The find() method returns the first element that satisfies a given condition let numbers = [10, 20, 30, 40]; let result = numbers.find(num => num > 25); console.log(result); // Output: 30 3. Using the filter() Method
If multiple elements match a condition, use filter() to return all matching values: let evenNumbers = numbers.filter(num => num % 2 === 0); console.log(evenNumbers); // Output: [10, 20, 30, 40] 4. Extracting Values with map()
The map() method transforms and retrieves values from an array: let doubled = numbers.map(num => num * 2); console.log(doubled); // Output: [20, 40, 60, 80] 5. Using Destructuring Assignment
You can use array destructuring to extract values easily: let [first, second] = fruits; console.log(first); // Output: Apple console.log(second); // Output: Banana 6. Using forEach() to Iterate Over an Array
The forEach() method helps in retrieving values while iterating: fruits.forEach(fruit => console.log(fruit)); 7. Using the reduce() Method
The reduce() method can be used to get a single value from an array let sum = numbers.reduce((acc, num) => acc + num, 0); console.log(sum); // Output: 100 Conclusion
JavaScript offers multiple ways to extract values from an array, each suitable for different use cases. Whether using simple indexing, higher-order functions like find(), map(), or reduce(), mastering these methods will help you efficiently handle arrays in JavaScript.
|