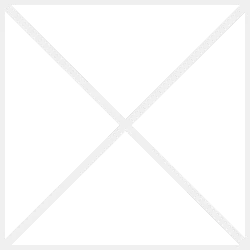
This ad doesn't have any photos.
|
In the world of programming, an array is one of the most fundamental and versatile data structures. Arrays allow developers to store multiple values in a single variable, making data management efficient and structured. Whether dealing with numbers, strings, or objects, an array of elements is a powerful tool used in almost every programming language. This article explores arrays, their types, applications, and advantages.
What is an Array? An array is a collection of elements stored in contiguous memory locations, enabling easy access and manipulation. Instead of declaring multiple individual variables, an array groups related elements under one name, simplifying data handling. Arrays can be one-dimensional, multidimensional, or associative, depending on how they are structured.
Types of Arrays One-Dimensional Arrays A one-dimensional array is the simplest form, consisting of a linear list of elements accessed via index values. Example in Python:
python Copy Edit numbers = [10, 20, 30, 40, 50] print(numbers[2]) # Output: 30 Multi-Dimensional Arrays These arrays contain multiple rows and columns, resembling a matrix. In Python, multi-dimensional arrays can be implemented using lists within lists or NumPy arrays:
python Copy Edit matrix = [[1, 2, 3], [4, 5, 6], [7, 8, 9]] print(matrix[1][2]) # Output: 6 Associative Arrays (Dictionaries in Python) While traditional arrays use numerical indices, associative arrays use key-value pairs for data storage. Example:
python Copy Edit student_scores = {"Alice": 85, "Bob": 90, "Charlie": 78} print(student_scores["Bob"]) # Output: 90 Applications of Arrays Data Storage: Used in databases and file systems for storing large datasets. Sorting & Searching: Algorithms like Bubble Sort, Quick Sort, and Binary Search rely on arrays. Game Development: Used for handling player inventories, maps, and game physics. Machine Learning & Data Science: Libraries like NumPy and Pandas heavily use arrays for numerical computations. Advantages of Arrays Efficient memory usage by storing elements in contiguous locations. Fast access to elements using index-based retrieval. Simplifies code by reducing the need for multiple variables. Conclusion An array of elements provides a structured way to store and manipulate data efficiently. Understanding arrays and their types helps programmers create optimized and scalable applications. Whether dealing with a simple list or complex multi-dimensional datasets, arrays remain a crucial part of programming logic.
|