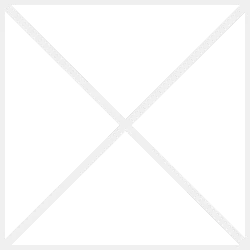
This ad doesn't have any photos.
|
javascript reduceright several array methods that help in manipulating and processing arrays effectively. One such method is reduceRight(), which is similar to reduce() but processes the array from right to left. This method is particularly useful in scenarios where you need to process elements in reverse order.
What is reduceRight()?
The reduceRight() method executes a reducer function on each element of an array, from right to left, resulting in a single accumulated value. It is useful when the order of processing matters, such as when dealing with concatenation, right-associative operations, or reducing expressions that require reverse execution.
Syntax: array.reduceRight(callback(accumulator, currentValue, index, array), initialValue) Parameters:
callback – A function that is executed on each element.
accumulator – The accumulated result from previous iterations.
currentValue – The current element being processed.
index – The index of the current element.
array – The array upon which reduceRight() is called.
initialValue (optional) – The initial value for the accumulator. If not provided, the last element of the array is used as the initial accumulator value.
Example Usage
Reversing String Concatenation
One of the common use cases of reduceRight() is concatenating strings in reverse order: const words = ["world!", " ", "Hello"]; const sentence = words.reduceRight((acc, curr) => acc + curr, ""); console.log(sentence); // Output: "Hello world!" Processing Numbers in Reverse Order
If we want to subtract elements of an array in a right-to-left manner: const numbers = [1, 2, 3, 4]; const result = numbers.reduceRight((acc, curr) => acc - curr); console.log(result); // Output: -2 (1 - (2 - (3 - 4))) Flattening an Array
reduceRight() can also be used to flatten a nested array: const nestedArray = [[3, 4], [1, 2]]; const flatArray = nestedArray.reduceRight((acc, curr) => acc.concat(curr), []); console.log(flatArray); // Output: [1, 2, 3, 4] Key Differences Between reduce() and reduceRight()
Order of Execution – reduce() iterates from left to right, while reduceRight() goes from right to left.
Use Cases – reduceRight() is preferable when dealing with expressions that are naturally right-associative, such as string concatenation or mathematical operations requiring reversed order.
Performance Considerations – Both methods have the same time complexity (O(n)), but reduceRight() may be slightly less intuitive when debugging.
When to Use reduceRight()
When order matters, such as reversing operations.
When working with right-associative expressions.
When processing nested arrays or objects in reverse.
Conclusion
reduceRight() is a powerful but often underutilized JavaScript method that provides great flexibility in array processing. By understanding its behavior and use cases, you can optimize operations that require right-to-left execution efficiently. While it may not be needed as frequently as reduce(), it’s a valuable tool for certain scenarios where order-dependent reductions are necessary.
|